Trong nhiệm vụ này, chúng tôi làm việc với các tệp. Các tập tin có ở khắp mọi nơi trong Vũ trụ này. Trong máy tính, tập tin hệ thống là một phần thiết yếu. Hệ điều hành bao gồm rất nhiều tệp.
Python có hai loại tệp-Tệp văn bản và tệp nhị phân.
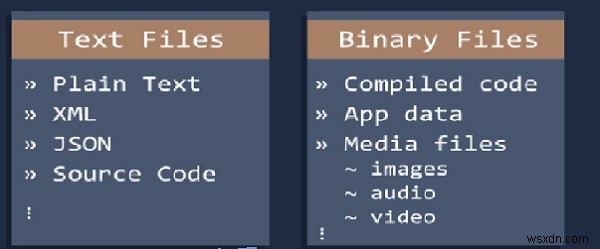
Ở đây chúng ta thảo luận về Tệp văn bản
Ở đây chúng tôi tập trung một số chức năng quan trọng trên tệp.
- Số lượng từ
- Số lượng ký tự
- Độ dài từ trung bình
- Số lượng từ dừng
- Số lượng ký tự đặc biệt
- Số lượng
- Số lượng các từ viết hoa
Chúng tôi có một tệp thử nghiệm "css3.txt", chúng tôi đang làm việc trên tệp đó
Số lượng từ
Khi chúng tôi đếm số từ trong một câu, chúng tôi sử dụng split hàm số. Đây là cách dễ nhất. Trong trường hợp này, chúng tôi cũng áp dụng chức năng phân tách.
Mã mẫu
filename="C:/Users/TP/Desktop/css3.txt" try: with open(filename) as file_object: contents=file_object.read() except FileNotFoundError: message="sorry" +filename print(message) else: words=contents.split() number_words=len(words) print("Total words of" + filename ,"is" , str(number_words))
Đầu ra
Total words of C:/Users/TP/Desktop/css3.txt is 3574
Số ký tự
Ở đây chúng tôi đếm số ký tự trong một từ, ở đây chúng tôi sử dụng độ dài của từ. Nếu độ dài là 5 thì từ đó có 5 ký tự.
Mã mẫu
filename="C:/Users/TP/Desktop/css3.txt" try: with open(filename) as file_object: contents=file_object.read() except FileNotFoundError: message="sorry" +filename print(message) else: words=0 characters=0 wordslist=contents.split() words+=len(wordslist) characters += sum(len(word) for word in wordslist) #print(lineno) print("TOTAL CHARACTERS IN A TEXT FILE =",characters)
Đầu ra
TOTAL CHARACTERS IN A TEXT FILE = 17783
Độ dài từ trung bình
Ở đây, chúng tôi tính tổng độ dài của tất cả các từ và chia nó cho tổng độ dài.
Mã mẫu
filename="C:/Users/TP/Desktop/css3.txt" try: with open(filename) as file_object: contents=file_object.read() except FileNotFoundError: message="sorry" +filename print(message) else: words=0 wordslist=contents.split() words=len(wordslist) average= sum(len(word) for word in wordslist)/words print("Average=",average)
Đầu ra
Average= 4.97
Số lượng từ dừng
Để giải quyết vấn đề này, chúng tôi sử dụng thư viện NLP trong Python.
Mã mẫu
from nltk.corpus import stopwords from nltk.tokenize import word_tokenize my_example_sent = "This is a sample sentence" mystop_words = set(stopwords.words('english')) my_word_tokens = word_tokenize(my_example_sent) my_filtered_sentence = [w for w in my_word_tokens if not w in mystop_words] my_filtered_sentence = [] for w in my_word_tokens: if w not in mystop_words: my_filtered_sentence.append(w) print(my_word_tokens) print(my_filtered_sentence)
Số ký tự đặc biệt
Tại đây, chúng ta có thể tính toán số lượng hashtag hoặc lượt đề cập có trong đó. Điều này giúp trích xuất thông tin bổ sung từ dữ liệu văn bản của chúng tôi.
Mã mẫu
import collections as ct filename="C:/Users/TP/Desktop/css3.txt" try: with open(filename) as file_object: contents=file_object.read() except FileNotFoundError: message="sorry" +filename print(message) else: words=contents.split() number_words=len(words) special_chars = "#" new=sum(v for k, v in ct.Counter(words).items() if k in special_chars) print("Total Special Characters", new)
Đầu ra
Total Special Characters 0
Số lượng
Ở đây chúng ta có thể tính toán số lượng dữ liệu số có trong các tệp văn bản. Nó giống như phép tính số ký tự trong một từ.
Mã mẫu
filename="C:/Users/TP/Desktop/css3.txt" try: with open(filename) as file_object: contents=file_object.read() except FileNotFoundError: message="sorry" +filename print(message) else: words=sum(map(str.isdigit, contents.split())) print("TOTAL NUMERIC IN A TEXT FILE =",words)
Đầu ra
TOTAL NUMERIC IN A TEXT FILE = 2
Số lượng từ viết hoa
Sử dụng hàm isupper (), chúng ta có thể tính toán số lượng các chữ cái viết hoa trong văn bản.
Mã mẫu
filename="C:/Users/TP/Desktop/css3.txt" try: with open(filename) as file_object: contents=file_object.read() except FileNotFoundError: message="sorry" +filename print(message) else: words=sum(map(str.isupper, contents.split())) print("TOTAL UPPERCASE WORDS IN A TEXT FILE =",words)
Đầu ra
TOTAL UPPERCASE WORDS IN A TEXT FILE = 121